In today's world, the exchange of data and information is an essential part of everyday life. With so much information being shared in different formats, it's important to know how to convert data from one format to another. In this blog post, we will be discussing how to convert JSON data to a PDF file using the iText library.
iText is a popular Java library for creating and manipulating PDF files. It provides a set of APIs for working with PDF documents, including text extraction, merging, and splitting PDF files, and creating PDF forms. It also supports the conversion of JSON data to PDF.
Prerequisites:
Before we dive into the process of converting JSON to PDF using the iText library, make sure that you have the following prerequisites in place:
- Java Development Kit (JDK) version 1.8 or later
- iText library version 5 or later
- A JSON file / or JSON String that you want to convert to PDF
- A basic understanding of Java programming
Steps to Convert JSON to PDF using iText: Now that we have the prerequisites in place, let's take a look at the steps involved in converting JSON data to a PDF file using the iText library.
Step 1: add maven dependencies
<dependency> <groupId>com.itextpdf</groupId> <artifactId>itextpdf</artifactId> <version>5.5.13.3</version> </dependency> <dependency> <groupId>com.google.code.gson</groupId> <artifactId>gson</artifactId> <version>3.10</version> </dependency>
Step 2: convert json string to JsonObject
Step 3: create iText pdf document with desired page size and path of output file location
Step 4: create PdfPtable
Step 5: populate data in to the table
Step 6: add the table to document
Step 7: close the document
package com.itext.jsontopdf; import com.google.gson.*; import com.itextpdf.text.*; import com.itextpdf.text.pdf.PdfPCell; import com.itextpdf.text.pdf.PdfPTable; import com.itextpdf.text.pdf.PdfWriter; import org.springframework.web.bind.annotation.*; import java.io.FileOutputStream; import java.util.Iterator; import java.util.Map; @RestController public class PDFController { @ResponseBody @PostMapping("/convertToPdf") public String convertJsonToPdf(@RequestBody String jsonData) { String msg = ""; try { //2. converting json string to jsonObject Gson gson = new Gson(); JsonParser parser = new JsonParser(); JsonArray array = parser.parse(jsonData).getAsJsonArray(); JsonObject object = new JsonObject(); object.add("item", array); JsonObject jsonObject = gson.fromJson(object, JsonObject.class); //3. create iText pdf document Document document = new Document(PageSize.A4); PdfWriter.getInstance(document, new FileOutputStream("/home/viveksoni/Documents" + "/jsontopdf.pdf")); document.open(); /** * we are taking the numOfColumn so we can use this ahead while declaring pdf table */ int numColumns = getNumOfColumns(jsonObject); //4. PdfPTable table = new PdfPTable(numColumns); Paragraph heading = new Paragraph("Student Data"); heading.setAlignment(Element.ALIGN_CENTER); document.add(heading); document.add(new Paragraph("\n")); //5. populate data in to the table // filling the header for (Map.Entry<String, JsonElement> entry : jsonObject.entrySet()) { for (JsonElement element : entry.getValue().getAsJsonArray()) { Iterator<Map.Entry<String, JsonElement>> iterator = ((JsonObject) element).entrySet().iterator(); while (iterator.hasNext()) { Font boldFont = new Font(Font.FontFamily.COURIER, 10, Font.BOLD); table.addCell(new PdfPCell(new Phrase(iterator.next().getKey(), boldFont))); } break; } } // filling the data for (Map.Entry<String, JsonElement> entry : jsonObject.entrySet()) { for (JsonElement element : entry.getValue().getAsJsonArray()) { Iterator<Map.Entry<String, JsonElement>> iterator = ((JsonObject) element).entrySet().iterator(); while (iterator.hasNext()) { Font normalFont = new Font(Font.FontFamily.COURIER, 9, Font.NORMAL); JsonElement jsonElement = iterator.next().getValue(); String value = ""; if (jsonElement != null && !jsonElement.isJsonNull()) { value = jsonElement.getAsString(); } Phrase phrase = new Phrase(value + " ", normalFont); PdfPCell cell = new PdfPCell(phrase); table.addCell(cell); } } } //6. add the table to the document document.add(table); //7. close the document document.close(); msg = "PDF conversion is successful"; } catch (Exception e) { e.printStackTrace(); msg = "PDF conversion failed due to : " + e.getMessage(); } return msg; } private int getNumOfColumns(JsonObject jsonObject) { int numColumns = 0; for (Map.Entry<String, JsonElement> entry : jsonObject.entrySet()) { for (JsonElement element : entry.getValue().getAsJsonArray()) { numColumns = ((JsonObject) element).entrySet().size(); break; } } return numColumns; } }
Step 8: test the application using postman
make a post request with the following url - http://localhost:8080/convertToPdf

write your json in the body section of the postman hit send
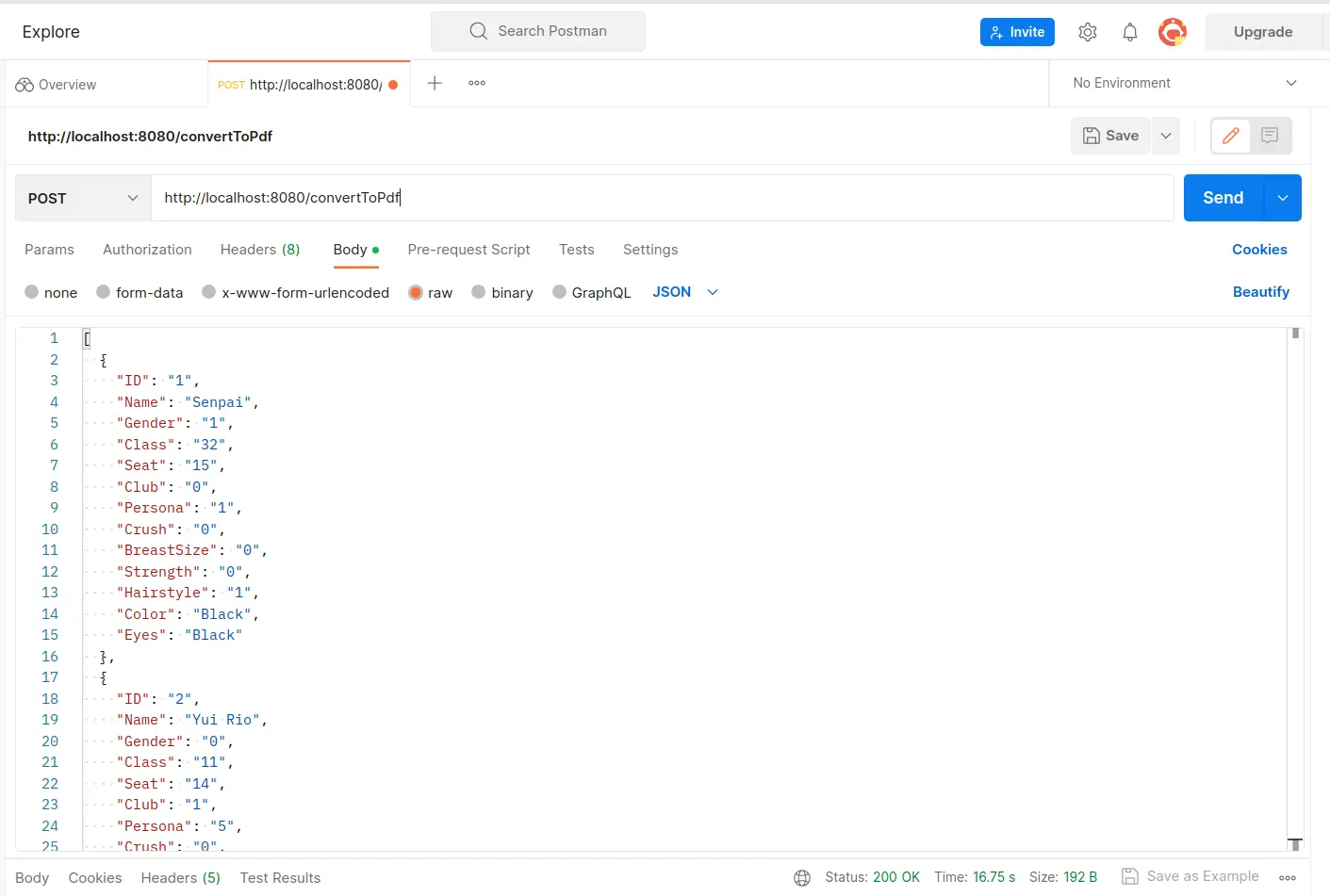
you will receive a 200 response and a file created on the provided path
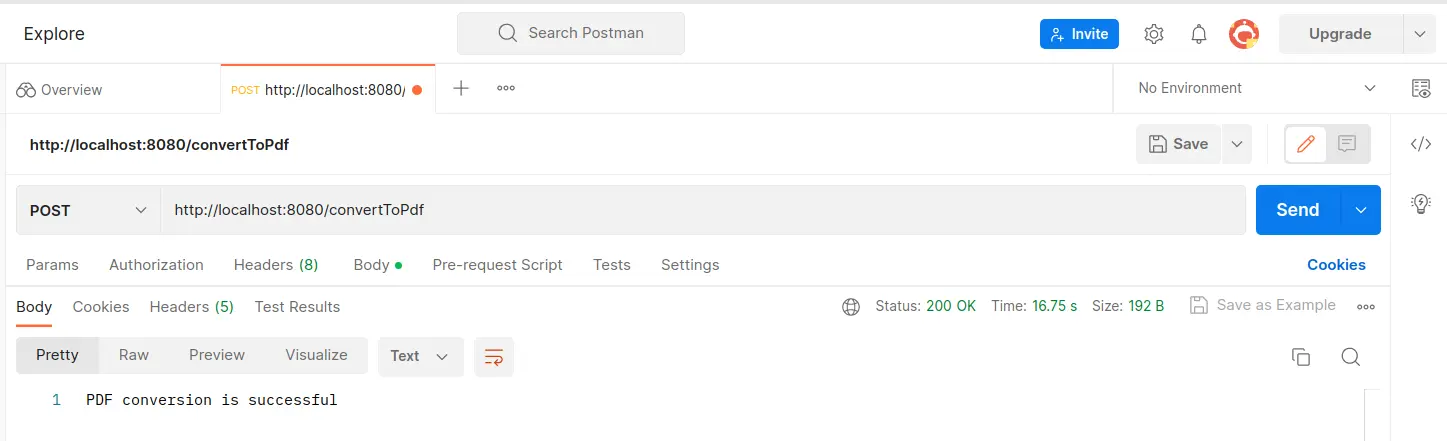

No comments:
Post a Comment